Kalman filter has a reputation for its difficulty. Invented by Rudolf E. Kalman in 1960, the Kalman filter is a mathematical technique widely used in Radar, navigation, and control systems. This filter was used in the famous Apollo program of NASA.
In the control system, navigation, and other measurement-related application, it is imperative to provide precise measurement and location of the subject. But in reality, in those cases, measurements got mixed with unknown noise, and hidden variables cause uncertain values and measurements. For instance, when we use GPS to navigate our smartphone, the time difference between the satellite signals is the measurement, and our velocity is a hidden parameter.
After knowing all these things, I started to look into this topic. As the undergrad syllabus doesn’t cover this topic, I had to look into youtube and luckily got one nice video.
So I thought to code a simple Kalman filter in python. In GitHub, there are numerous code implementations given already. But for me, I need to build everything from scratch to understand the core concept. Some implementations used the OOP approach. I have taken two approaches to build a simple one-dimensional Kalman Filter. The first one is a manual method where, after every iteration, the values will be used for the next iteration. But in this way, after 3 blocks of code, I felt I need to automate this thing, so I make a for loop, and now everything in one block of code.
The equations are
\begin{aligned} Kalman\ Gain = \frac{Error\ in \ Estimate} {Error\ in \ Estimate \ + \ Error \ in \ Measurement} \end {aligned}
\begin{aligned} New \ Estimate = {Previous \ Estimate} \ + \ {Kalman \ Gain \ X (Measurement \ -\ Previous \ Estimate )} \end {aligned}
\begin{aligned} Updated \ Error\ in \ Estimate = {[1- Kalman\ Gain]} \ X \ {\ Previous \ Error\ in\ Estimate )} \end {aligned}
Example 1:
- Initial Estimate = 68
- Measurement = 75, 71, 70
- Initial Error In Estimate = 2
- Error in Measurement = 4 [fixed]
Resultant Estimate = 70.33333333333333, 70.5, 70.4090909090909
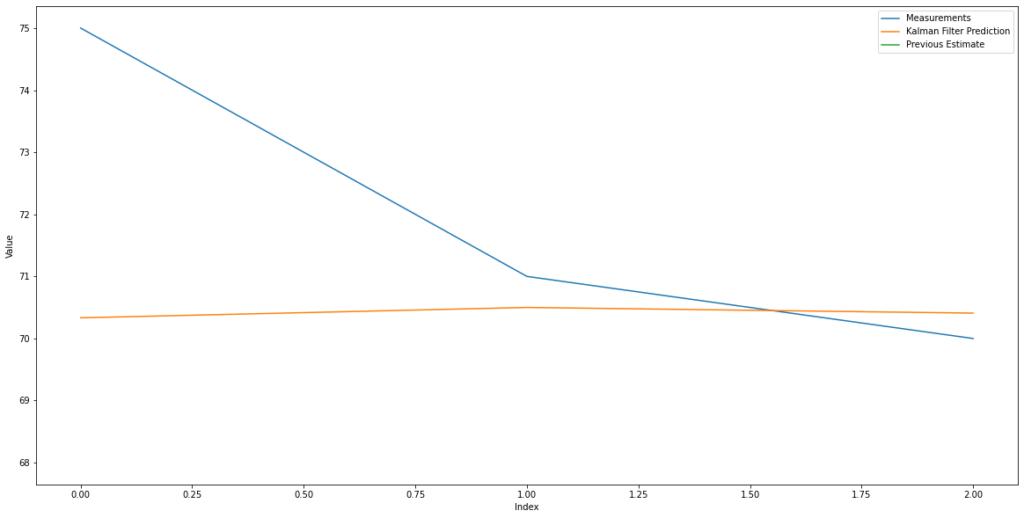
Example 2:
- Initial Estimate = 68
- Measurement = [73, 79, 76, 74, 77, 71, 73, 73, 71, 79, 75, 74, 75, 75, 75, 77, 77, 70, 78, 72, 74, 77, 73, 73, 70, 74, 71, 78, 78, 74, 73, 70, 72, 73, 76, 70, 70, 74, 75, 77, 73, 78, 70, 71, 79, 71, 72, 78, 72, 71]
- Initial Error In Estimate = 2
- Error in Measurement = 6 [fixed]
Resultant Estimate: [69.25, 71.2, 72.0, 72.28571428571429, 72.875, 72.66666666666667, 72.7, 72.72727272727273, 72.58333333333334, 73.07692307692308, 73.21428571428572, 73.26666666666668, 73.37500000000001, 73.47058823529413, 73.55555555555557, 73.73684210526318, 73.90000000000002, 73.71428571428574, 73.90909090909093, 73.82608695652176, 73.83333333333336, 73.96000000000002, 73.92307692307695, 73.88888888888891, 73.75000000000003, 73.7586206896552, 73.6666666666667, 73.80645161290326, 73.93750000000003, 73.93939393939397, 73.91176470588238, 73.80000000000003, 73.75000000000003, 73.72972972972975, 73.78947368421055, 73.69230769230771, 73.60000000000001, 73.60975609756099, 73.64285714285715, 73.72093023255815, 73.70454545454547, 73.80000000000001, 73.71739130434784, 73.65957446808513, 73.77083333333336, 73.71428571428574, 73.68000000000002, 73.76470588235296, 73.73076923076924, 73.67924528301887]
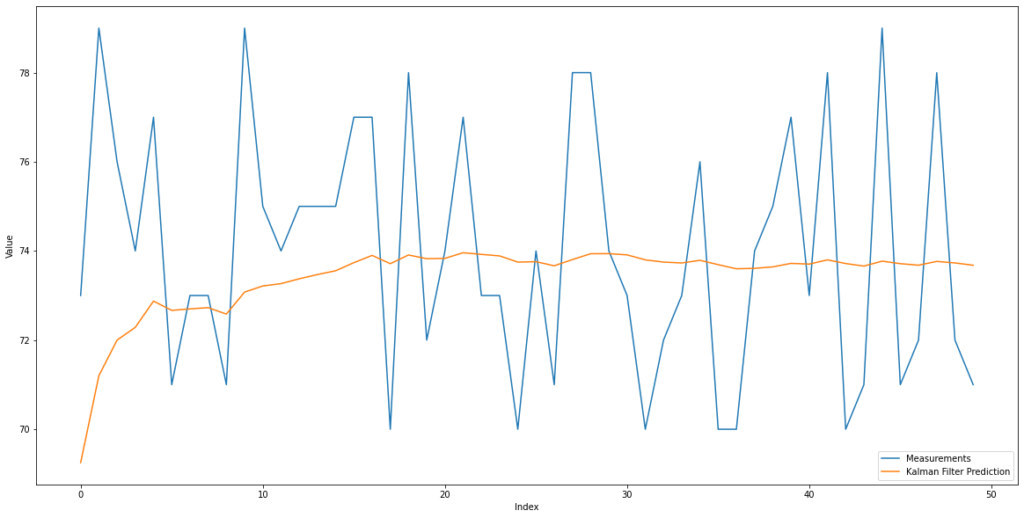